how to create a loop in python
This article was published as a part of the Data Science Blogathon
Overview:
This article is a walkthrough of several types of loops and control statements with plenf practice exercises. So, I advise you to read the concepts and practice the examples along with me. Let's start.
Table of contents:
- Loops and their importance
- Loop Types
-
- while loop
- for loop
- nested loop
-
- Loop Control statements
- break statement
- continue statement
- pass statement
Loops and their importance
In a programming language, a loop is a statement that contains instructions that continually repeats until a certain condition is reached.
Loops help us remove the redundancy of code when a task has to be repeated several times. With the use of loops, we can cut short those hundred lines of code to a few. Suppose you want to print the text "Hello, World!" 10 times. Rather than writing a print statement 10 times, you can make use of loops by indicating the number of repetitions needed.
Image by Author (Made using Microsoft Whiteboard)
Loop Types
The three types of loops in Python programming are:
- while loop
- for loop
- nested loops
while loop
It continually executes the statements(code) as long as the given condition is TRUE. It first checks the condition and then jumps into the instructions.
Syntax:
while condition: statements(code)
Inside the while loop, we can have any number of statements. The condition may be anything as per our requirement. The loop stops running when the condition fails (become false), and the execution will move to the next line of code.
Flow Diagram of while loop
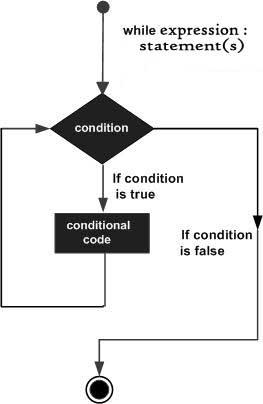
It first checks the condition, executes the conditional code if the condition is TRUE, and checks the condition again. Program control exits the loop if the condition is FALSE.
Example 1: Print the text "Hello, World!" 5 times.
num_of_times = 1 while num_of_times <= 5: print("Hello, World!") num_of_times += 1
Explanation: The loop runs as long as the num_of_times variable is less than or equal to 5. num_of_times increments by 1 after each iteration.
(if you are a beginner, use the Thonny IDE to see the step-by-step execution)
Output:
Example 2: Create a list of all the even numbers between 1 and 10
num = 1 even_numbers = [] while num <= 10: if num % 2 == 0: even_numbers .append(num) num += 1 print("Even Numbers list: ", even_numbers )
Explanation: The loop runs as long as the num variable is less than or equal to 10. If the condition is TRUE, the program control enters the loop and appends the num to the even_numbers list if the number is cleanly divisible by 2.
Output:
Example 3: Creating an infinite loop
A loop runs infinite times when the condition never fails.
i = True while i: print("Condition satisfied")
Output:
Example 4: use else with a while loop
When an else statement is used along with a while loop, the control goes to the else statement when the condition is False.
- var = 1
- while var <= 4:
- print(f"Condition is TRUE: {var} <= 4″)
- var += 1
- else:
- print(f"Condition is FALSE: {var} > 4″)
Output:
for loop
A for loop is used to iterate over a sequence like lists, type, dictionaries, sets, or even strings.
Loop statements will be executed for each item of the sequence.
Syntax of for loop:
for item in iterator: statements(code)
Flow diagram of for loop:
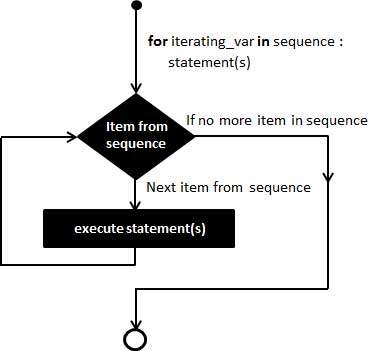
Takes the first item of the iterable, executes the statement, and moves the pointer to the next item until it reaches the last item of the sequence.
Example 1: Print the text "Hello, World!" 5 times.
list = [1, 2, 3, 4, 5] for num in list: print("Hello, World!")
the variable num is not used in the code, so we can use the below syntax (use underscore):
list = [1, 2, 3, 4, 5] for _ in list: print("Hello, World!")
(using the range function to return a sequence of numbers from 1 to 10. Read more about it from here)
even_nums = [] for i in range(1, 11): if i % 2 == 0: even_nums.append(i) print("Even Numbers: ", even_nums)
Example 3: Creating an infinite loop
An infinite loop can be created using a loop by appending a new element to the list after every iteration.
num = [0] for i in num: print(i) num.append(i+1)
Output:
Example 4: use else with a for loop
iterator = (1, 2, 3, 4) for item in iterator: print(item) else: print("No more items in the iterator")
Output:
Example 5: Display the items of a dictionary
example = { 'iterator': 'dictionary', 'loop': 'for', 'operation': 'display dictionary elements' } for key in example: print(f"{key}: {example[key]}")
The key, value of a dictionary can be directly accessed using .items()
for key, value in example.items(): print(f"{key}: {value}")
Output:
Nested loops
Nested loops mean using a loop inside another loop. We can use any type of loop inside any type of loop. We can use a while loop inside for loop, a for loop inside a while loop, a while loop inside a while loop, or a for loop inside a for loop.
Example: Create a list of even numbers between 1 and 10
even_list = [] for item in range(1, 11): while item % 2 == 0: even_list.append(item) break print("Even Numbers: ", even_list)
Output:
Loop Control Statements
Loop control statements are used to change the flow of execution. These can be used if you wish to skip an iteration or stop the execution.
The three types of loop control statements are:
- break statement
- continue statement
- pass statement
break statement
based on the given condition, the break statement stops the execution and brings the control out of the loop.
Example: Create a list of the odd numbers between 1 and 20 (use while, break)
num = 1 odd_nums = [] while num: if num % 2 != 0: odd_nums.append(num) if num >=20: break num += 1 print("Odd numbers: ", odd_nums)
Example: Stop the execution if the current number is 5 (use for, break)
for num in range(1, 11): if num == 5: break else: print(num)Continue statement
Continue statement is used to skip the current iteration when the condition is met and allows the loop to continue with the next iteration. It does not bring the control out of the loop unline the break statement.
Example: Skip the iteration if the current number is 6 (use while, continue)
num = 0 while num < 10: num += 1 if num == 6: continue print(num)
Example: Skip the iteration if the current number is 6 (use for, continue)
for num in range(1, 11): if num == 6: continue print(num)
Pass Statement
Pass statement is used when we want to do nothing when the condition is met. It doesn't skip or stop the execution, it just passes to the next iteration. Sometimes we use comment which is ignored by the interpreter. Pass is not ignored and can be used with loops, functions, classes, etc.
It is useful when we do not want to write functionality at present but want to implement it in the future.
Example: while, pass statement
num = 1 while num <= 10: if num == 6: pass print(num) num += 1
Example: for, pass statement
for num in range(1, 11): if num == 6: pass print(num)
As an exercise, run the code snippets and see how the control statements work.
End Notes:
I am glad you are determined to read till the conclusion. By the end of this article, we are familiar with the types of loops, control statements in Python.
I hope this article is informative. Feel free to share it with your study buddies.
References:
Fork the complete code file from the GitHub repo
Other Blog Posts by me
Feel free to check out my other blog posts from my Analytics Vidhya Profile.
You can find me on LinkedIn, Twitter in case you would want to connect. I would be glad to connect with you.
For immediate exchange of thoughts, please write to me at [email protected].
Happy Learning!
Image 1 – https://www.tutorialspoint.com/python/python_loops.htm
Image 2 – https://www.tutorialspoint.com/python/python_for_loop.htm
The media shown in this article are not owned by Analytics Vidhya and are used at the Author's discretion.
how to create a loop in python
Source: https://www.analyticsvidhya.com/blog/2021/09/loops-and-control-statements-an-in-depth-python-tutorial/
Posted by: andersonbarives.blogspot.com
0 Response to "how to create a loop in python"
Post a Comment